So, you’ve got your WordPress site humming along on a Bitnami instance in the cloud on aws ec2 instance, and maybe you are utilizing the aws free tier benefits for 1 year of free wordpress hosting. Awesome! But then you hit a snag: your site isn’t sending emails. Maybe you’ve got a contact form that’s silently failing, or perhaps you’re trying to send notifications from a custom script. Whatever the reason, you need your PHP mail()
function to actually work.
You might have stumbled upon plugins like “WP Mail SMTP” and those are great for many situations. But what if you need something more fundamental, something that works even outside of WordPress, directly at the server level? That’s where msmtp
comes into play. Think of it as a tiny, efficient mailman that sits on your server and handles email delivery.
This guide will walk you through Getting Your Bitnami WordPress on AWS to Send Emails: A No-Plugin Guide (Using MSMTP). We’ll cover installation, configuration, troubleshooting, and even testing. We’re aiming for that satisfying feeling when you finally see that “Email sent successfully” message!
Why MSMTP? The “Why” Before the “How”
Before we dive into the technical steps, let’s understand why we’re choosing msmtp
over, say, a WordPress plugin:
- Beyond WordPress: WP Mail SMTP, and similar plugins, are fantastic for configuring WordPress-specific email settings (like routing emails through Gmail). But they rely on WordPress itself being up and running. If you have other PHP scripts (maybe a custom cron job, a data processing script, etc.) that need to send emails independently of WordPress, a plugin won’t cut it.
msmtp
operates at the system level, making it available to any PHP script. - Lightweight and Focused:
msmtp
is designed to do one thing and do it well: send emails via SMTP. It doesn’t have the overhead of a full-fledged mail server. This makes it a great choice for instances where you just need basic email sending functionality without overloading constrained resources on your aws server. - Direct
mail()
Function Support: The goal here is to get PHP’s built-inmail()
function working.msmtp
seamlessly integrates withmail()
, so you don’t have to rewrite your existing code.
Table of Contents
How to configure Gmail SMTP on Bitnami AWS WordPress Instance to send Emails
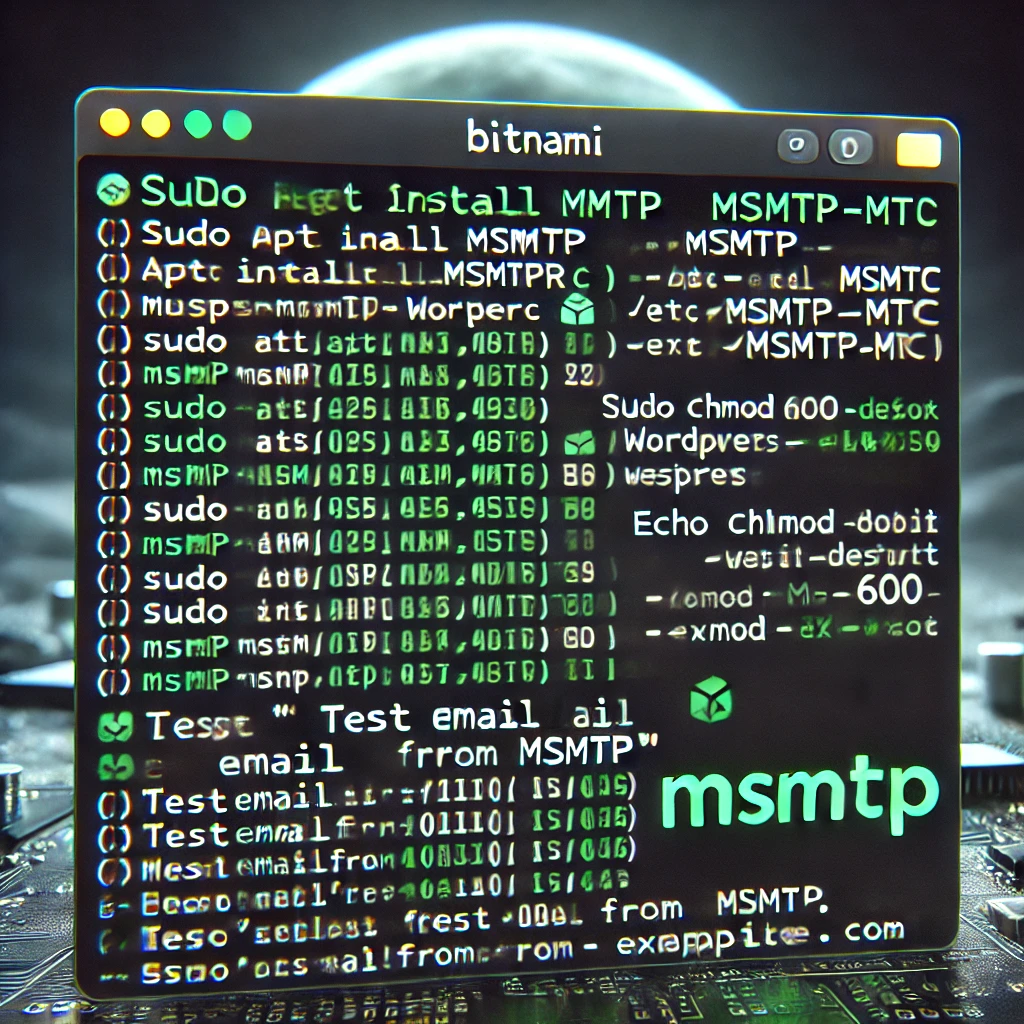
Step 1: Installing MSMTP – Getting the Mailman on Board
First things first, let’s get msmtp
installed. You’ll need to connect to your Bitnami instance via SSH. Use your favorite ssh terminal, like Putty, or others to do so.
Once you’re connected, run these commands:
sudo apt-get update
sudo apt-get install msmtp msmtp-mta
During the installation, you might see a question about “AppArmor support.” For most setups, choosing No is perfectly fine and simplifies things. If you’re running a high-security environment and understand AppArmor, you can choose Yes, but it might require extra configuration.
Step 2: Configuring MSMTP – Telling It Where to Deliver the Mail
Now that msmtp
is installed, we need to tell it how to send emails. This involves creating a configuration file. We’ll use the system-wide configuration, which is generally the best approach.
- Open the configuration file:
sudo nano /etc/msmtprc
(We’re usingnano
here, a simple text editor. Feel free to usevim
or another editor if you’re comfortable with them.) - Enter your configuration: Paste the following into the file, but make sure to replace the placeholders with your actual Gmail information:
defaults
auth on
tls on
tls_trust_file /etc/ssl/certs/ca-certificates.crt
logfile /var/log/msmtp.log
account default
host smtp.gmail.com
port 587
from [email protected]
user [email protected]
password your-gmail-app-password
- Save the file: In
nano
, pressCTRL + X
, thenY
(to confirm saving), and thenENTER
. - Set Permissions – Keeping Things Secure: This is a very important step. We need to make sure the configuration file is only readable by the user that needs it and not by everyone on the system.
sudo chmod 600 /etc/msmtprc
sudo chown bitnami:daemon /etc/msmtprc
sudo touch /var/log/msmtp.log
sudo chmod 666 /var/log/msmtp.log
sudo chown bitnami:daemon /var/log/msmtp.log
The chmod 600
command means “only the owner can read and write.”
The chown bitnami:daemon
command sets the owner to the bitnami
user and the group to daemon
. This is Bitnami’s recommended practice, as PHP-FPM runs as daemon.
Step 3: Testing – Let’s Send an Email!
Before we integrate this with PHP, let’s make sure msmtp
itself is working. Run this command:
echo "This is a test email from msmtp!" | msmtp --debug --from=default -t [email protected]
Replace [email protected]
with a real email address where you can receive the test message.
The --debug
flag will show you a lot of detailed output. If everything is configured correctly, you should see messages like:
Resolving host smtp.gmail.com...
Connecting to smtp.gmail.com:587...
TLS handshake successful...
SMTP status: 250 2.1.0 OK
And, most importantly, you should receive the email at the recipient address! If you get errors, carefully review the troubleshooting steps above and check the debug output for clues.
Step 4: Troubleshooting – Because Things Don’t Always Go Smoothly
Let’s be realistic: sometimes, things don’t work perfectly the first time. Here are some common errors you might encounter and how to fix them:
- Error:
msmtp: account default not found: no configuration file available
Fix: Double-check your/etc/msmtprc
file. Make sure you only have oneaccount default
section. If you accidentally pasted the configuration twice, remove the duplicate. - Error:
Ignoring system configuration file /etc/msmtprc: Permission denied
Fix: This means the permissions on the configuration file are incorrect. Run thechmod
andchown
commands from Step 2 again to ensure they’re set correctly. Permissions being the most common errors. - Error:
msmtp: cannot log to /var/log/msmtp.log: cannot open: Permission denied
Fix: Themsmtp
process doesn’t have permission to write to the log file. Fix it:- Option 1 (Easier): Give
msmtp
permission to the existing log file:sudo touch /var/log/msmtp.log # Create the file if it doesn't exist sudo chmod 666 /var/log/msmtp.log # Allow everyone to read and write (less secure) sudo chown bitnami:bitnami /var/log/msmtp.log # Set ownership
- Option 1 (Easier): Give
Step 5: Integrating with PHP – Making mail()
Work
Now for the final step: telling PHP to use msmtp
.
- Open the PHP configuration file:
sudo nano /opt/bitnami/php/etc/php.ini
- Find the
sendmail_path
: Search for a line that starts withsendmail_path
. It might be commented out (with a semicolon at the beginning). - Modify the line: Change the line to:
sendmail_path = "/usr/bin/msmtp -t"
If the line had a semicolon, remove it i.e. uncomment it. - Restart: This is crucial for the changes to take effect:
sudo /opt/bitnami/ctlscript.sh restart
That’s it! Now, any PHP script on your server that uses the mail()
function will automatically send emails through msmtp
, using your Gmail account.
Testing with a PHP Script
Create a simple PHP file (e.g., testmail.php
) in your web directory:
<?php
$to = "[email protected]"; // Replace with your recipient
$subject = "Test Email from PHP";
$message = "This is a test email sent using PHP's mail() function and msmtp.";
$headers = "From: [email protected]"; // Replace with your Gmail
if (mail($to, $subject, $message, $headers)) {
echo "Email sent successfully!";
} else {
echo "Email sending failed.";
}
?>
Browse this php file and the output should be shown.
Limitations of this Method
While msmtp
is a great solution for many cases, it’s important to be aware of its limitations:
- Sending Only:
msmtp
is a send-only mail transfer agent. It cannot receive emails or manage mailboxes. It’s purely for sending outgoing messages. - SMTP Relay:
msmtp
relies on an external SMTP server (like Gmail’s in this case). You’re bound by the sending limits and policies of that provider. Gmail, for example, has daily sending limits. - Spam Filters: Emails sent through a relay service like Gmail might be more likely to end up in spam folders, especially if your server’s IP address doesn’t have a good reputation. Properly configuring SPF, DKIM, and DMARC records for your domain can help mitigate this (but that’s a topic for another guide!).
- Security: While we’ve set permissions securely, always be mindful of storing credentials. If your server is compromised, the Gmail App Password could be exposed so we strongly recommend creating a separate email address for this usecase.
- Error Handling: The PHP
mail()
function’s return value (true/false) doesn’t provide detailed error information. If sending fails, you’ll need to check themsmtp
log file to diagnose the problem. Consider using a more robust PHP mail library (like PHPMailer) for better error handling and features if you need more control. - Gmail Specific: This guide focuses on using Gmail. If you want to use a different SMTP provider, you’ll need to adjust the
host
,port
, and potentially the authentication settings in themsmtprc
file.
You’ve Got Mail!
I hope this guide helped you sucessfully set up msmtp
on your Bitnami WordPress instance, and your PHP mail()
function should now be working reliably. Feel free to ask any questions in comments below.